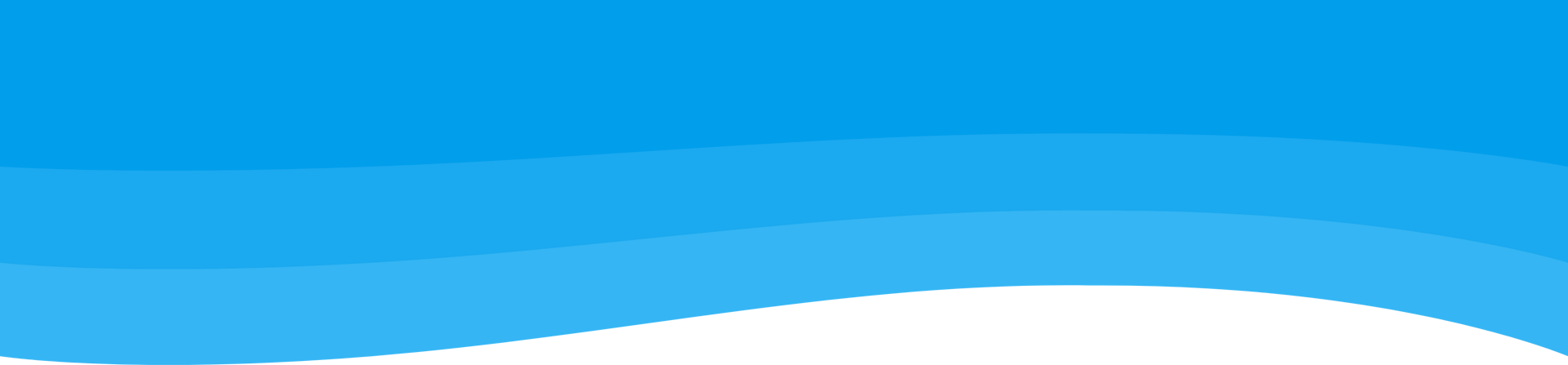
Inventive
Insights
The Role of Content Marketing in SEO
In this guide, we'll delve deeper into the relationship between content marketing and SEO and outline common SEO content marketing strategies.
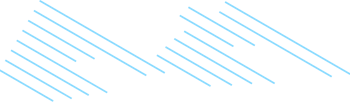
In this guide, we'll delve deeper into the relationship between content marketing and SEO and outline common SEO content marketing strategies.